이번에는 옵션 메뉴(Option Menu)를 사용하는 실습을 해 봅시다.
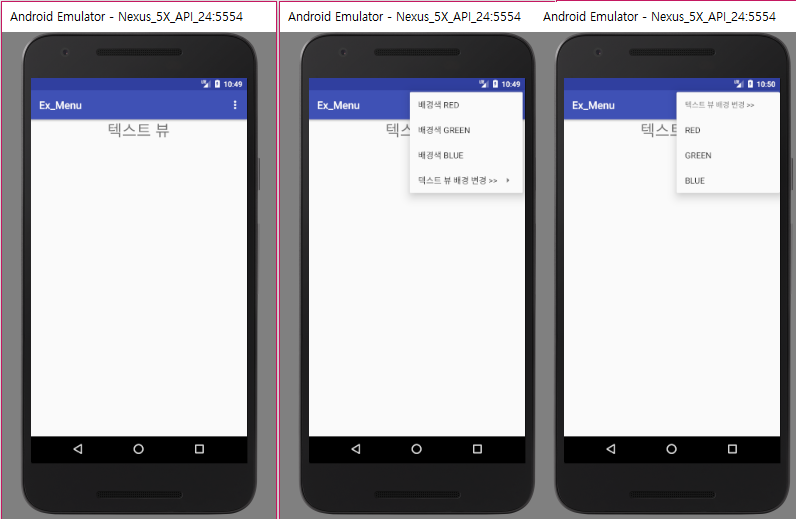
먼저 프로젝트 뷰의 app>>res 하위에 menu 폴더를 추가하고 menu1.xml 파일을 추가하세요.
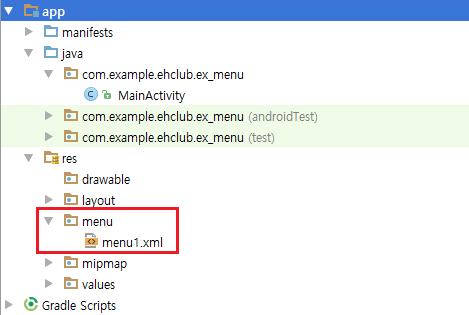
다음처럼 메뉴를 작성합시다.
<?xml version="1.0" encoding="utf-8"?> <menu xmlns:android="http://schemas.android.com/apk/res/android"> <item android:id="@+id/item_red" android:title="배경색 RED"/> <item android:id="@+id/item_green" android:title="배경색 GREEN"/> <item android:id="@+id/item_blue" android:title="배경색 BLUE"/> <item android:title="텍스트 뷰 배경 변경 >>" > <menu> <item android:id="@+id/item_tvred" android:title="RED"/> <item android:id="@+id/item_tvgreen" android:title="GREEN"/> <item android:id="@+id/item_tvblue" android:title="BLUE"/> </menu> </item> </menu>
activity_main.xml은 다음처럼 작성하세요. 여기에서는 단순히 메뉴에서 전체 배경 색상과 텍스트 뷰의 배경 색상을 변경할 것이기 때문에 id를 부여하는 작업 정도면 충분합니다.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.example.ehclub.ex_menu.MainActivity" android:orientation="vertical" android:id="@+id/root_layout"> <TextView android:id="@+id/tv" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="30sp" android:textAlignment="center" android:text="텍스트 뷰" /> </LinearLayout>
이제 MainActivity.java 소스 파일을 편집합시다. 먼저 두 개의 컨트롤을 참조할 수 있는 멤버 필드를 선언하세요.
LinearLayout root_layout; TextView tv;
onCreate 메서드에서는 두 개의 멤버 필드가 컨트롤을 참조합니다.
root_layout = (LinearLayout)findViewById(R.id.root_layout); tv = (TextView) findViewById(R.id.tv);
onCreateOptionMenu 메서드를 재정의하세요. 안드로이드 스튜디오의 인텔리센스를 통해 자동 완성을 이용하면 편합니다. 먼저 MenuInflater 개체를 얻어온 후에 inflate 메서드를 통해 메뉴를 설정합니다.
@Override public boolean onCreateOptionsMenu(Menu menu) { super.onCreateOptionsMenu(menu); MenuInflater mi = getMenuInflater(); mi.inflate(R.menu.menu1,menu); return true; }
onOptionsItemSelected 메서드를 재정의합니다. 여기에서는 어떠한 메뉴 항목을 선택하였는지에 따라 적절한 처리 구문을 작성합니다. 이번 실습에서는 선택한 메뉴 항목에 따라 최상위 layout의 배경색을 변경하거나 TextView의 배경색을 변경합시다.
@Override public boolean onOptionsItemSelected(MenuItem item) { switch (item.getItemId()){ case R.id.item_red: root_layout.setBackgroundColor(Color.RED); return true; case R.id.item_green: root_layout.setBackgroundColor(Color.GREEN); return true; case R.id.item_blue: root_layout.setBackgroundColor(Color.BLUE); return true; case R.id.item_tvred: tv.setBackgroundColor(Color.RED); return true; case R.id.item_tvgreen: tv.setBackgroundColor(Color.GREEN); return true; case R.id.item_tvblue: tv.setBackgroundColor(Color.BLUE); return true; } return false; }
다음은 MainActivity.java 파일의 소스 코드입니다.
package com.example.ehclub.ex_menu; import android.graphics.Color; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.Menu; import android.view.MenuInflater; import android.view.MenuItem; import android.widget.LinearLayout; import android.widget.TextView; public class MainActivity extends AppCompatActivity { LinearLayout root_layout; TextView tv; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); root_layout = (LinearLayout)findViewById(R.id.root_layout); tv = (TextView) findViewById(R.id.tv); } @Override public boolean onCreateOptionsMenu(Menu menu) { super.onCreateOptionsMenu(menu); MenuInflater mi = getMenuInflater(); mi.inflate(R.menu.menu1,menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { switch (item.getItemId()){ case R.id.item_red: root_layout.setBackgroundColor(Color.RED); return true; case R.id.item_green: root_layout.setBackgroundColor(Color.GREEN); return true; case R.id.item_blue: root_layout.setBackgroundColor(Color.BLUE); return true; case R.id.item_tvred: tv.setBackgroundColor(Color.RED); return true; case R.id.item_tvgreen: tv.setBackgroundColor(Color.GREEN); return true; case R.id.item_tvblue: tv.setBackgroundColor(Color.BLUE); return true; } return false; } }