이번에는 On, Off 상태를 설정할 때 사용하는 Switch 컨트롤을 이용하는 간단한 앱을 만들어 봅시다.
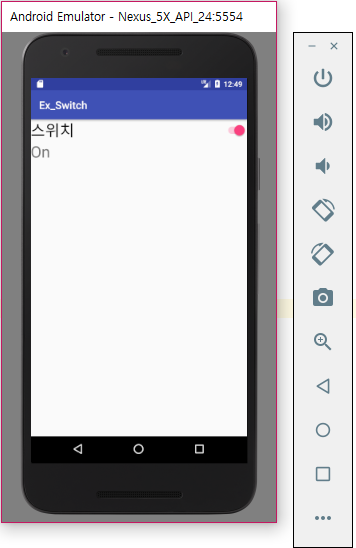
먼저 activity_main.xml에 컨트롤을 배치합시다. 최상위 요소는 LinearLayout을 배치합니다. 그리고 자식으로 Switch와 TextView를 배치하세요.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.example.ehclub.ex_switch.MainActivity" android:orientation="vertical"> <Switch android:layout_width="match_parent" android:layout_height="wrap_content" android:text="스위치" android:textSize="30sp" android:id="@+id/sw" /> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="[상태]" android:textSize="30sp" android:id="@+id/tv"/> </LinearLayout>
이제 MainActivity.java 파일을 편집합시다. 먼저 Switch 형식의 멤버 필드를 선언하세요.
Switch sw;
onCreate 메서드에서 findViewById 메서드를 호출하여 xml에 배치한 Switch를 참조합니다.
sw = (Switch)findViewById(R.id.sw);
초기 Switch 상태에 따라 TextView를 설정하는 작업을 호출합시다. 이 부분은 별도의 메서드로 작성할 것입니다.
CheckState();
스위치의 클릭 리스너를 설정하고 리스너에서는 Switch 상태에 따라 TextView를 설정하는 CheckState 메서드를 호출합니다.
sw.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { CheckState(); } });
이제 CheckState 메서드를 작성합시다. 스위치의 체크 상태는 isChecked 메서드를 호출하면 알 수 있습니다. 이를 이용하여 TextView의 text 속성을 설정합니다.
private void CheckState(){ TextView tv = (TextView)findViewById(R.id.tv); if(sw.isChecked()) { tv.setText("On"); } else{ tv.setText("Off"); } }
다음은 MainActivity.java 파일의 내용입니다.
package com.example.ehclub.ex_switch; import android.graphics.Color; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Switch; import android.widget.TextView; public class MainActivity extends AppCompatActivity { Switch sw; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); sw = (Switch)findViewById(R.id.sw); CheckState(); sw.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { CheckState(); } }); } private void CheckState(){ TextView tv = (TextView)findViewById(R.id.tv); if(sw.isChecked()) { tv.setText("On"); } else{ tv.setText("Off"); } } }