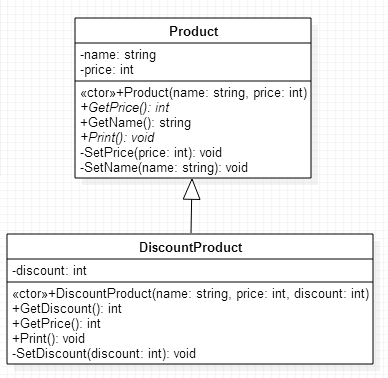
이제 상속과 다형성 실습을 해 봅시다. 이번 실습은 상품을 소재로 할게요.
시나리오
- 상품
상품 이름과 가격을 멤버 필드로 갖습니다.
생성할 때 이름과 가격을 입력 인자로 받습니다.
가격과 이름의 접근자를 제공하며 가격 접근자는 가상 메서드입니다.
상품 정보를 출력하는 가상 메서드를 제공합니다.
형식 내부에서만 접근 가능한 가격 설정자와 이름 설정자가 있습니다.
- 할인 상품
할인율을 멤버 필드로 갖습니다.
상품 이름과 가격, 할인율을 입력 인자로 받습니다.
가격 접근자와 상품 정보 출력하는 메서드를 재정의합니다.
할인율의 접근자 메서드를 제공합니다.
형식 내부에서만 접근할 수 있는 할인율 설장자가 있습니다.
여러분께서 먼저 작성해 본 후에 비교해 보세요. 작성하다 막히면 앞에 상속과 다형성에 관한 내용을 보시면서 하시기 바랍니다. 여기에 작성한 것을 보면서 그대로 따라하는 것은 큰 의미가 없어요.
다음은 예제 코드입니다.
//상속과 다형성 실습1(상품과 할인 상품) #include <string> #include <iostream> using namespace std; class Product { string name; int price; public: Product(string name,int price) //생성자 { SetName(name); SetPrice(price); } virtual int GetPrice()const //가격접근자 가상 메서드 { return price; } string GetName()const //이름접근자 { return name; } virtual void Print()const//정보 출력 가상 메서드 { cout<<name<<" 판매가격: "<<GetPrice()<<endl; } private: void SetPrice(int price) //가격설정자 { this->price = price; } void SetName(string name) //이름설정자 { this->name = name; } }; class DiscountProduct: public Product { int discount; public: DiscountProduct(string name,int price,int discount) :Product(name,price) //기반 클래스에 기본 생성자를 제공하지 않을 때 초기화 구문 사용 { SetDiscount(discount); } int GetDiscount()const //할인율 접근자 { return discount; } virtual int GetPrice()const //가격 접근자 재정의 { int origin_price = Product::GetPrice(); int dc = origin_price * discount/100; return origin_price - dc; } virtual void Print()const //정보 출력 메서드 재정의 { cout<<"상품가격:"<<Product::GetPrice()<<" 할인율:"<<discount<<" "; Product::Print(); } private: void SetDiscount(int discount) //할인율 설정자 { this->discount = discount; } }; int main (void) { Product *p1 = new Product("치약",3000); Product *p2 = new DiscountProduct("칫솔",3000,15); p1->Print(); p2->Print(); delete p1; delete p2; return 0; }
▷ 실행 결과
치약 판매가격: 3000
상품가격:3000 할인율:15 칫솔 판매가격: 2550