캡슐화 실습을 해 보기로 해요. 이번에는 복소수 클래스 캡슐화예요.
OOP 프로그래밍 할 때 구현 이전의 작업과 이후의 많은 작업을 CASE 도구로 UML로 표현할 때가 많아요. CASE 도구에는 Rose, Together, StarUML 등이 있습니다.
이 책에서는 CASE 도구를 사용하는 방법을 구체적으로 다루지는 않지만 많은 곳에서 UML로 작성한 다이어그램으로 실습할 내용 등을 소개할 거예요.
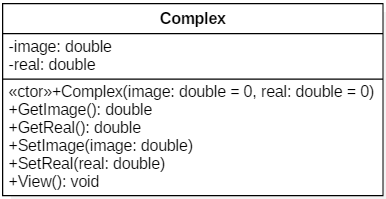
UML로 클래스를 표현할 때는 세 칸으로 구성한 사각형으로 나타내요. 맨 위는 클래스 이름, 두 번째는 멤버 필드, 마지막 칸은 멤버 메서드를 표현합니다.
멤버 앞에 +(public), -(private), #(protected)은 접근 지정자를 나타내죠. 그리고 멤버 필드명 뒤에 콜론(:)이 오고 콜론 뒤에 형식을 표현해요.
특수한 멤버나 클래스에 << >>로 감싸는 것을 스테레오라고 부르며 여기에서 ctor은 생성자를 나타내기 위해 개인적으로 표현한 것입니다.
멤버 메서드에서드 뒤에 콜론 뒤에 오는 것이 반환 형식입니다.
이번에는 실수부와 허수부로 구성하는 복소수를 Complex 이름으로 정의하기로 해요.
먼저 클래스를 프로젝트에 추가해야겠죠.
class Complex//복소수 클래스 { 그리고 클래스 다이어그램 두 번째 칸에 표현한 멤버 필드를 클래스 정의문에 선언하세요. //멤버 필드(멤버 변수) double image; double real; 그리고 멤버 메서드들을 추가하세요. 현재 클래스 다이어그램에서는 모든 멤버 메서드는 public으로 지정하였으므로 접근 지정자를 먼저 표시합시다. public: 그리고 멤버 메서드를 추가하세요. Complex(double real=0, double image=0);//생성자 멤버 필드의 값을 제공하는 메서드를 접근자라고 부르며 설정하는 메서드를 설정자라고 부릅니다. 일반적으로 접근자는 Get으로 시작하고 설정자는 Set으로 시작해요. double GetImage()const;//허수 접근자(가져오기) double GetReal()const;//실수 접근자(가져오기) void SetImage(double image);//허수 설정자(설정하기) void SetReal(double real);//실수 설정자(설정하기) void View()const;//정보 출력 };
헤더 파일에 클래스를 정의하였으면 이제 소스 파일에 구체적으로 정의하세요.
생성자에서는 입력 인자로 받은 값으로 멤버 필드를 설정해야죠. 멤버 메서드로 설정자가 있으니 이를 호출해서 설정하기로 해요.
Complex::Complex(double real, double image) { SetReal(real); SetImage(image); }
접근자 메서드에서는 멤버 필드를 반환하세요.
double Complex::GetImage()const { return image; } double Complex::GetReal()const { return real; }
설정자에서는 입력 인자로 받은 값으로 멤버 필드를 설정하세요.
void Complex::SetImage(double image) { this->image = image; } void Complex::SetReal(double real) { this->real = real; }
정보 출력하는 메서드를 구현합시다. 실수부와 허수부의 값에 따라 어떻게 출력할 것인지 잘 생각하시고 사용자가 이해하기 편하게 출력하게 구현하세요.
void Complex::View()const { if((real!=0)&&(image != 0))//실수부, 허수부 모두 0이 아닐 때 { cout<<real<<"+"<<image<<"i"<<endl; } else //실수부나 허수부 중에 최소 하나는 0일 때 { if(image!=0)//허수부가 0이 아닐 때(실수부는 0) { cout<<image<<"i"<<endl; } else//허수부가 0일 때 { cout<<real<<endl; } } }
마지막으로 집입점 main 함수를 작성하세요. 진입점이 있는 소스 파일은 Program.cpp로 정할게요. 여러분께서는 다양한 인자를 사용하여 복소수 개체를 생성한 후 값을 출력해 보세요.
int main() { Complex *c1 = new Complex(); Complex *c2 = new Complex(2.1); Complex *c3 = new Complex(2.1,3.3); Complex *c4 = new Complex(0,3.3); Complex *c5 = new Complex(2.1,0); Complex *c6 = new Complex(2.1,-3.3); c1->View(); c2->View(); c3->View(); c4->View(); c5->View(); c6->View(); delete c1; delete c2; delete c3; delete c4; delete c5; delete c6; return 0; }
다음은 이번 실습의 전체 내용입니다.
//Complex.h #pragma once #include <string> #include <iostream> using namespace std; class Complex//복소수 클래스 { //멤버 필드(멤버 변수) double image; double real; public: Complex(double real=0, double image=0);//생성자 double GetImage()const;//허수 접근자(가져오기) double GetReal()const;//실수 접근자(가져오기) void SetImage(double image);//허수 설정자(설정하기) void SetReal(double real);//실수 설정자(설정하기) void View()const;//정보 출력 };
//Complex.cpp #include "Complex.h" Complex::Complex(double real, double image) { SetReal(real); SetImage(image); } double Complex::GetImage()const { return image; } double Complex::GetReal()const { return real; } void Complex::SetImage(double image) { this->image = image; } void Complex::SetReal(double real) { this->real = real; } void Complex::View()const { if((real!=0)&&(image != 0))//실수부, 허수부 모두 0이 아닐 때 { cout<<real<<"+"<<image<<"i"<<endl; } else //실수부나 허수부 중에 최소 하나는 0일 때 { if(image!=0)//허수부가 0이 아닐 때(실수부는 0) { cout<<image<<"i"<<endl; } else//허수부가 0일 때 { cout<<real<<endl; } } }
//Program.cpp #include "Complex.h" int main() { Complex *c1 = new Complex(); Complex *c2 = new Complex(2.1); Complex *c3 = new Complex(2.1,3.3); Complex *c4 = new Complex(0,3.3); Complex *c5 = new Complex(2.1,0); Complex *c6 = new Complex(2.1,-3.3); c1->View(); c2->View(); c3->View(); c4->View(); c5->View(); c6->View(); delete c1; delete c2; delete c3; delete c4; delete c5; delete c6; return 0; }
▷ 실행 결과
0
2.1
2.1+3.3i
3.3i
2.1
2.1+-3.3i
여러분께서는 보다 사용자가 편하게 정의해 보세요.